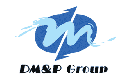 |
DM&P Serial Port DOS Library Reference |
Serial Port Library
SerPort DOS library is from the kernel of our RSIP that supports 8 serial ports and interrupt-driven communication. It provides simple C functions and save a lot of development time for programmer. SerPort is for DM&P products with M6117D/Vortex86/Vortex86SX CPUs. It is a DOS real mode and large memory model library.
Before using SerPort library, you should know the I/O base address and IRQ of COM ports you want to use. The default IRQ of COM1/2 is IRQ4/3. If SBC has 4 COM ports, the factory default value is that COM3/4 uses the same IRQ as COM1/2.The default I/O base address and IRQ of our SBC are:
COM1 |
3F8H |
IRQ4 |
COM2 |
2F8H |
IRQ3 |
COM3 |
3E8H |
IRQ4 |
COM4 |
2E8H |
IRQ3 |
For 4 COM ports usage, we recommend user to set COM3/4 to use IRQ10/11 because SerPort does not support shared IRQ.For example, you can find a JP7 of 6026 to set COM3/4 to use IRQ10/11.The table will show you the IRQ usage SerPort supports:
COM1 |
COM2 |
COM3 |
COM4 |
Status |
IRQ4 |
IRQ3 |
|
|
OK |
|
|
IRQ4 |
IRQ3 |
OK |
IRQ4 |
IRQ3 |
IRQ10 |
IRQ11 |
OK |
IRQ4 |
IRQ3 |
IRQ4 |
IRQ3 |
Not Supported |
For 8 COM ports usage, we recommend those configurations::
COM Port |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
IRQ |
4 |
3 |
10 |
11 |
5 |
6 |
7 |
15 |
I/O Address |
3F8H |
2F8H |
3E8H |
2E8H |
338H |
238H |
228H |
220H |
P.S. Make sure the IRQ in table is not used by other PCI device. This is because SerPort does not support shared IRQ.
User can find a demo.c from SerPort library. It is a demo program that use COM1 to send key press messages and use COM2 to receive messages. You should have a crossed cable (pin2 and pin 3 is changed) before your test. Connect COM1 and COM2 on your SBC with crossed cable. Run demo.exe, any key press will send to COM1 and COM2 will receive the message. Or, you can connect COM1 of SBC(1) to COM2 of SBC(2). Both of then run demo.exe and any key press message on SBC(1) will send to SBC(2).
Function Reference
int SerPort_Open(enum COM_t ComPort,unsigned int nBufSize,unsigned int nBaseAddr,unsigned int nIrq);
Description: |
Initialize COM port and set buffer size. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
nBufSize |
the buffer size for COM port. |
nBaseAddr |
I/O base address, refer to default table below. |
nIrq |
the IRQ you want to use. |
COM1 |
3F8H |
IRQ4 |
COM2 |
2F8H |
IRQ3 |
COM3 |
3E8H |
IRQ4 |
COM4 |
2E8H |
IRQ3 |
|
Example: |
SerPort_Open(COM1,4096,0x3f8,4); |
int SerPort_Close(enum COM_t ComPort);
Description: |
Close a COM port. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
|
Example: |
SerPort_Close(COM1); |
int SerPort_IsOk(enum COM_t ComPort);
Description: |
Detect COM port is okay or not. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
|
Example: |
if(SerPort_IsOk(COM1)==0) printf("Unable to initialize COM1.\n"); |
void SerPort_SetParam(enum COM_t ComPort,enum Baud_t Baud,enum Parity_t Parity,enum Length_t Length,enum StopBit_t StopBit);
Description: |
Set COM port parameters. |
Arguments: |
Return |
N/A |
ComPort |
Pre-defined values: COM1~COM8. |
Baud |
Pre-defined values: BAUD_50, BAUD_75, BAUD_110, BAUD_300, BAUD_1200, BAUD_2400, BAUD_4800, BAUD_9600, BAUD_14400, BAUD_19200, BAUD_38400, BAUD_57600, BAUD_115200. |
Parity |
Pre-defined values: PARITY_NO, PARITY_ODD, PARITY_EVEN, PARITY_MARK, PARITY_Space. |
Length |
Pre-defined values: LENGTH_5_BIT, LENGTH_6_BIT, LENGTH_7_BIT, LENGTH_8_BIT. |
StopBit |
Pre-defined values: STOPBIT_1_BIT, STOPBIT_2_BIT. |
|
Example: |
/* Set baud rate 115200 bps, no parity, 8 bits, 1 stop bit */SerPort_SetParam(COM1,BAUD_115200,PARITY_NO,LENGTH_8_BIT,STOPBIT_1_BIT); |
void SerPort_Clear(enum COM_t ComPort);
Description: |
Clear data in buffer. |
Arguments: |
Return |
N/A |
ComPort |
Pre-defined values: COM1~COM8. |
|
Example: |
SerPort_Clear(COM1); |
int SerPort_IsParityError(enum COM_t ComPort);
Description: |
Detect parity error. |
Arguments: |
Return |
N/A |
ComPort |
Pre-defined values: COM1~COM8. |
|
Example: |
if(SerPort_IsParityError(COM1)!=0) printf("Parity error.\n"); |
unsigned int SerPort_Avail(enum COM_t ComPort);
Description: |
Get size of available data in buffer. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
|
Example: |
if(SerPort_Avail(COM1)) SerPort_RecvBuf(COM1,pcBuf,1024); |
int SerPort_SendBuf(enum COM_t ComPort,const char *pcBuf,unsigned int nSize);
Description: |
Send a buffer to COM port. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
pcBuf |
Buffer pointer. |
nSize |
Buffer size. |
|
Example: |
SerPort_SendBuf(COM1,pcBuf,1024); |
int SerPort_SendStr(enum COM_t ComPort,const char *szStr);
Description: |
Send a ASCIIZ string to COM port. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
szStr |
ASCIIZ pointer. |
|
Example: |
char *szAtCmd = "ATZ";SerPort_SendStr(COM1,szAtCmd); |
int SerPort_SendByte(enum COM_t ComPort,char c);
Description: |
Send one byte to COM port. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
c |
Byte to send. |
|
Example: |
char c = 'x';SerPort_SendByte(COM1,c); |
int SerPort_RecvBuf(enum COM_t ComPort,char *pcBuf,unsigned int nSize);
Description: |
Receive data from COM port. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
pcBuf |
Buffer pointer. |
nSize |
Buffer size. |
|
Example: |
char szBuf[1024];SerPort(COM1,szBuf,1024); |
int SerPort_RecvByte(enum COM_t ComPort,char *pc);
Description: |
Receive one byte from COM port. |
Arguments: |
Return |
0 is error, non-zero is okay. |
ComPort |
Pre-defined values: COM1~COM8. |
pc |
Buffer to receive one byte. |
|
Example: |
char c;SerPort_RecvByte(COM1,&c); |
int SerPort_GetDTR(enum COM_t ComPort);
Description: |
Get data terminal ready status. |
Arguments: |
Return |
0 is unset, 1 is set. |
ComPort |
Pre-defined values: COM1~COM8. |
|
Example: |
if(SerPort_GetDTR(COM1)){/* do something */} |
void SerPort_SetDTR(enum COM_t ComPort,int n);
Description: |
Set data terminal ready status. |
Arguments: |
Return |
N/A |
ComPort |
Pre-defined values: COM1~COM8. |
n |
Value to set DTR status |
|
Example: |
SerPort_SetDTR(COM1,1); |
int SerPort_GetRTS(enum COM_t ComPort);
Description: |
Get request to send status. |
Arguments: |
Return |
0 is unset, 1 is set. |
ComPort |
Pre-defined values: COM1~COM8. |
|
Example: |
if(SerPort_GetRTS(COM1)){/* do something */} |
void SerPort_SetRTS(enum COM_t ComPort,int n);
Description: |
Set request to send status. |
Arguments: |
Return |
N/A |
ComPort |
Pre-defined values: COM1~COM8. |
n |
Value to set RTS status |
|
Example: |
SerPort_SetRTS(COM1,1); |